Understanding Hash Tables: Implementation and Best Practices
Hash tables are a fundamental data structure in computer science, providing efficient data retrieval. In this post, we’ll walk you through the basics of hash tables, why they’re important, and how to implement them using direct chaining with linked lists. Whether you’re a beginner or an experienced developer, this guide will help you understand and use hash tables effectively.
🌟 Special Offer for My Readers 🌟
Use code:
LEARNWITHME
at checkout on Udemy to get 90% off my courses.The Complete Data Structures and Algorithms in Python
Complete Python Bootcamp For Everyone From Zero to Hero
Python Database Course: SQLite, PostgreSQL, MySQL,SQLAlchemy
What is a Hash Table?
A hash table, or hash map, is a data structure that maps keys to values. It uses a hash function to compute an index into an array of buckets or slots, from which the desired value can be found.
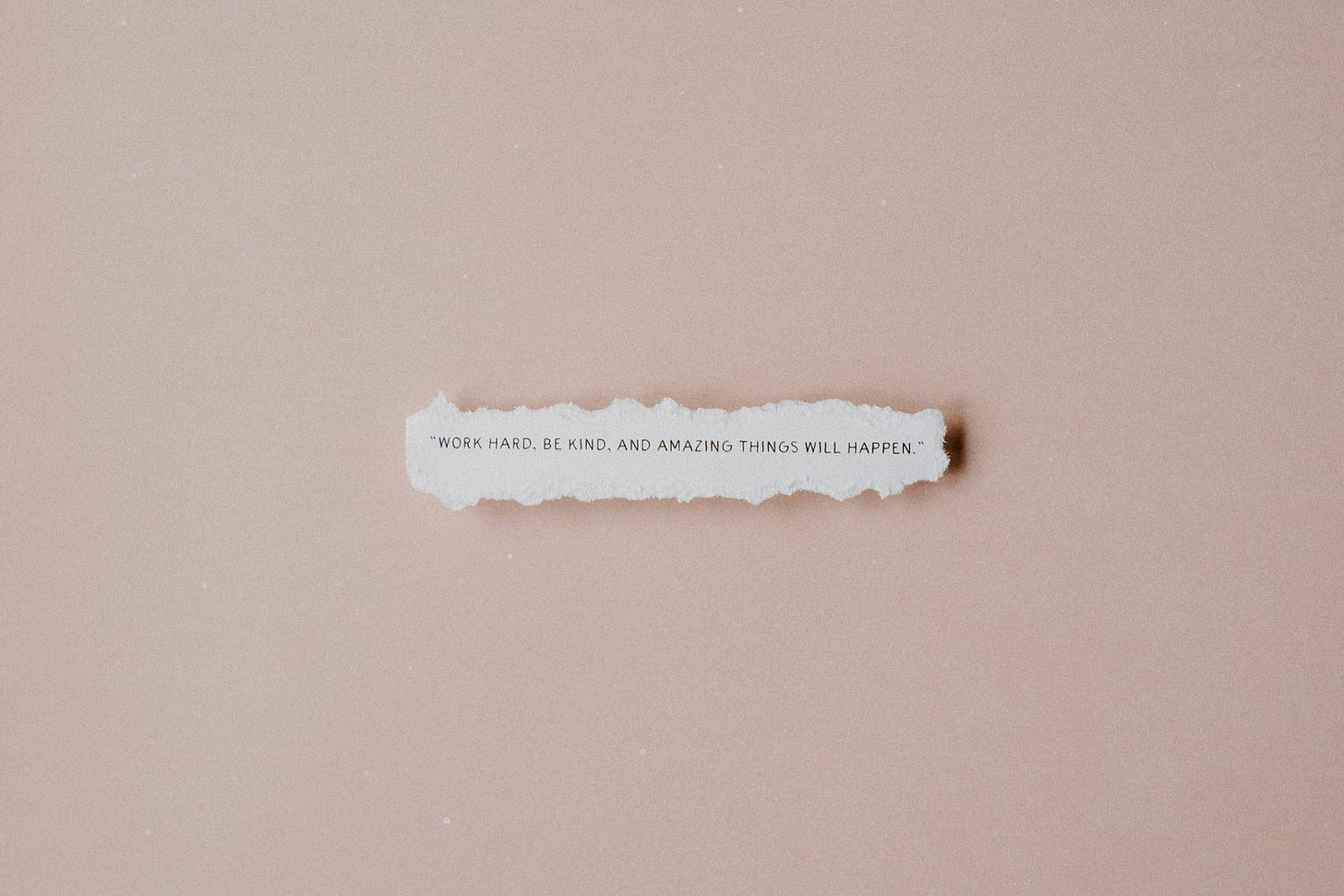
Why Use Hash Tables?
Hash tables are known for their efficiency, offering average-case time complexity of O(1) for lookups, insertions, and deletions. This makes them ideal for applications where you need fast data retrieval.
Basic Concepts
Key-Value Pairs
Hash tables store data in key-value pairs. The key is a unique identifier for the data, and the value is the data itself.
Hash Function
A hash function converts a given key into an index in the array. A good hash function distributes keys uniformly across the array to minimize collisions.
Collisions
A collision occurs when two keys hash to the same index. Collisions are handled using techniques like chaining or open addressing. In this guide, we’ll use chaining with linked lists.
Implementation
Let’s implement a simple hash table in Python using direct chaining with linked lists.
Step 1: Define the Hash Function
First, we need a hash function to convert keys into array indices. Python’s built-in hash()
function is a good starting point.
def hash_function(key, size):
return hash(key) % size
Step 2: Create the Hash Table Class
Next, we’ll create a HashTable
class to manage our hash table.
class HashTable:
def __init__(self, size):
self.size = size
self.table = [[] for _ in range(size)]
In the constructor, we initialize an array of empty lists. Each list will act as a bucket to handle collisions.
Step 3: Implement the Insert Method
The insert
method adds a key-value pair to the hash table.
def insert(self, key, value):
index = hash_function(key, self.size)
# Check if the key already exists
for pair in self.table[index]:
if pair[0] == key:
pair[1] = value # Update the existing value
return
# If the key does not exist, add a new pair
self.table[index].append([key, value])Copy code
Step 4: Implement the Retrieve Method
The retrieve
method fetches the value associated with a given key.
def retrieve(self, key):
index = hash_function(key, self.size)
for pair in self.table[index]:
if pair[0] == key:
return pair[1]
return None # Key not foundCopy code
Step 5: Implement the Delete Method
The delete
method removes a key-value pair from the hash table.
def delete(self, key):
index = hash_function(key, self.size)
for i, pair in enumerate(self.table[index]):
if pair[0] == key:
del self.table[index][i]
return
Complete Hash Table Class
Here is the complete HashTable
class with all methods:
class HashTable:
def __init__(self, size):
self.size = size
self.table = [[] for _ in range(size)]
def hash_function(self, key):
return hash(key) % self.size
def insert(self, key, value):
index = self.hash_function(key)
for pair in self.table[index]:
if pair[0] == key:
pair[1] = value # Update the existing value
return
self.table[index].append([key, value])
def retrieve(self, key):
index = self.hash_function(key)
for pair in self.table[index]:
if pair[0] == key:
return pair[1]
return None # Key not found
def delete(self, key):
index = self.hash_function(key)
for i, pair in enumerate(self.table[index]):
if pair[0] == key:
del self.table[index][i]
return
Example Usage
Now, let’s see how to use our HashTable
class.
# Create a hash table
hash_table = HashTable(10)
# Insert key-value pairs
hash_table.insert("name", "Alice")
hash_table.insert("age", 30)
hash_table.insert("city", "New York")
# Retrieve values
print(hash_table.retrieve("name")) # Output: Alice
print(hash_table.retrieve("age")) # Output: 30
print(hash_table.retrieve("city")) # Output: New York
# Delete a key-value pair
hash_table.delete("age")
print(hash_table.retrieve("age")) # Output: None
Best Practices
Choosing a Good Hash Function
A good hash function should distribute keys uniformly across the array to minimize collisions. Python’s built-in hash()
function is generally sufficient, but you may need a custom hash function for specific use cases.
Handling Collisions
Using chaining with linked lists, as shown in our implementation, is one way to handle collisions. Another method is open addressing, where you probe for the next available slot.
Resize Strategy
To maintain performance, consider implementing dynamic resizing of the hash table when the load factor (number of elements divided by the array size) exceeds a certain threshold. This involves creating a larger array and rehashing all existing keys.
Hash tables are a powerful tool in any developer’s toolkit. Understanding their implementation and best practices will help you write more efficient and effective code. By following this guide, you should now have a solid understanding of how to implement a basic hash table using direct chaining with linked lists.
Feel free to experiment with the code and try different scenarios to deepen your understanding. Happy coding!
If you found them helpful:
Discover more in my online courses at AppMillers 🚀
Connect on LinkedIn: Elshad Karimov
Follow on X (Twitter): Elshad Karimov
Subscribe to our Newsletter for recent Technological advancements: Newsletter by Elshad Karimov